Conway-Markdown is:
the filenames would look like Windows executables from the 90s.
#placeholder-markers
#literals
#display-code
#comments
#divisions
#blockquotes
#unordered-lists
#ordered-lists
#tables
#headings
#paragraphs
#inline-code
#boilerplate
#boilerplate-properties
#cmd-properties
#boilerplate-protect
#backslash-escapes
#backslash-continuations
#reference-definitions
#specified-images
#referenced-images
#explicit-links
#specified-links
#referenced-links
#inline-semantics
#escape-idle-html
#whitespace
#placeholder-unprotect
#placeholder-protect
#de-indent
#escape-html
#trim-whitespace
#reduce-whitespace
#code-tag-wrap
#prepend-newline
#unordered-list-items
#ordered-list-items
#mark-table-headers-for-preceding-table-data
#table-headers
#table-data
#unmark-table-headers-for-preceding-table-data
#table-rows
#table-head
#table-body
#table-foot
#suppress-scheme
#angle-bracket-wrap
ReplacementSequence
PlaceholderMarkerReplacement
PlaceholderProtectionReplacement
PlaceholderUnprotectionReplacement
DeIndentationReplacement
OrdinaryDictionaryReplacement
RegexDictionaryReplacement
FixedDelimitersReplacement
ExtensibleFenceReplacement
PartitioningReplacement
InlineAssortedDelimitersReplacement
HeadingReplacement
ReferenceDefinitionReplacement
SpecifiedImageReplacement
ReferencedImageReplacement
ExplicitLinkReplacement
SpecifiedLinkReplacement
ReferencedLinkReplacement
Conway-Markdown is published to PyPI as conwaymd
:
$ pip3 install conwaymd
pipx
instead of pip3
to avoid having to set up a virtual environment.
pip
instead of pip3
.
$ cmd [-h] [-v] [-a] [-x] [file.cmd ...]
Convert Conway-Markdown (CMD) to HTML.
positional arguments:
file.cmd name of CMD file to be converted (can be abbreviated as
`file` or `file.` for increased productivity)
options:
-h, --help show this help message and exit
-v, --version show program's version number and exit
-a, --all convert all CMD files under the working directory
-x, --verbose run in verbose mode (prints every replacement applied)
On Windows:
cmd-
or conwaymd
instead of cmd
to avoid summoning Command Prompt.
.cmd
files by accident;
they might break your computer. God save!
Code:
from conwaymd.core import cmd_to_html
cmd_content = '''
# Test
==
- This is a *near*-minimal test.
- Here be [__dragons__].
==
[__dragons__]: https://example.com/
'''
html_content = cmd_to_html(cmd_content, cmd_file_name='scripting-test.py')
print(html_content)
Output:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>Title</title>
</head>
<body>
<h1>Test</h1>
<ul>
<li>
This is a <em>near</em>-minimal test.
</li>
<li>
Here be <a href="https://example.com/"><b>dragons</b></a>.
</li>
</ul>
</body>
</html>
CMD files are parsed thus:
«replacement rules»
«delimiter»
«main content»
«replacement rules»
are user-defined CMD replacement rules
that will be used in addition to the standard CMD replacement rules.
«delimiter»
is the first occurrence of
3-or-more percent signs on its own line.
(If no «delimiter»
is found in the file,
the whole file is parsed as «main content»
.)
«main content»
is the CMD content
that will be converted to HTML according to
the standard and user-defined CMD replacement rules.
In the implementation:
STANDARD_RULES
in constants.py
are parsed,
and CMD replacement rules are added to the replacement queue accordingly.
«replacement rules»
in the CMD file are parsed,
and CMD replacement rules are added or inserted into the replacement queue
accordingly.
«main content»
to convert it to HTML.
To get started with writing «main content»
,
read the listing of standard CMD replacement rules.
To learn about writing user-defined «replacement rules»
,
read about CMD replacement rule syntax.
This section lists the standard CMD replacement rules
as defined by the constant string STANDARD_RULES
in constants.py
.
Some replacement rules are queued, in that they appear explicitly in the replacement queue.
Other replacement rules are unqueued, in that they do not appear explicitly in the replacement queue. However, they might be called by queued replacements.
#placeholder-markers
PlaceholderMarkerReplacement: #placeholder-markers
- queue_position: ROOT
Replaces occurrences of the placeholder marker «U+F8FF»
with a placeholder
so that the occurrences will not be confounding.
#literals
ExtensibleFenceReplacement: #literals
- queue_position: AFTER #placeholder-markers
- syntax_type: INLINE
- allowed_flags:
u=KEEP_HTML_UNESCAPED
i=KEEP_INDENTED
w=REDUCE_WHITESPACE
- prologue_delimiter: <
- extensible_delimiter: `
- content_replacements:
#escape-html
#de-indent
#trim-whitespace
#reduce-whitespace
#placeholder-protect
- epilogue_delimiter: >
<` «content» `>
«flags»<` «content» `>
«flags»
:
u
: keep HTML unescaped (do not apply #escape-html
)
i
: keep indented (do not apply #de-indent
)
w
: reduce whitespace (apply #reduce-whitespace
)
`
may be increased arbitrarily.
Preserves «content»
literally.
<` <b>Foo</b> `>
<b>Foo</b>
u<` <b>Foo</b> `>
<b>Foo</b>
#literals
syntax itself:
<`` Literally <`literal`>. ``>
Literally <`literal`>.
#display-code
ExtensibleFenceReplacement: #display-code
- queue_position: AFTER #literals
- syntax_type: BLOCK
- allowed_flags:
u=KEEP_HTML_UNESCAPED
i=KEEP_INDENTED
w=REDUCE_WHITESPACE
- extensible_delimiter: ``
- attribute_specifications: EMPTY
- content_replacements:
#escape-html
#de-indent
#reduce-whitespace
#code-tag-wrap
#placeholder-protect
- tag_name: pre
``
«content»
``
``{«attribute specifications»}
«content»
``
«flags»``
«content»
``
«flags»``{«attribute specifications»}
«content»
``
«flags»
:
u
: keep HTML unescaped (do not apply #escape-html
)
i
: keep indented (do not apply #de-indent
)
w
: reduce whitespace (apply #reduce-whitespace
)
`
may be increased arbitrarily.
«attribute specifications»
:
see CMD attribute specifications.
Produces (pre-formatted) display code:
<pre«attribute sequence»><code>«content»
</code></pre>
``{.java}
for (int index = 0; index < count; index++)
{
// etc. etc.
}
``
<pre class="java"><code>for (int index = 0; index < count; index++)
{
// etc. etc.
}
</code></pre>
for (int index = 0; index < count; index++)
{
// etc. etc.
}
#literals
with flag u
to inject HTML:
``
Injection of <b> element u<` <b>here</b> `>.
``
<pre><code>Injection of <b> element <b>here</b>.
</code></pre>
Injection of <b> element here.
#comments
RegexDictionaryReplacement: #comments
- queue_position: AFTER #display-code
* [^\S\n]*
[<]
(?P<hashes> [#]+ )
[\s\S]*?
(?P=hashes)
[>]
-->
<# «content» #>
#
may be increased arbitrarily.
Remove CMD comments, along with all preceding whitespace.
#display-code
prevails over #comments
:
``
Before.
<# This be a comment. #>
After.
``
<pre><code>Before.
<# This be a comment. #>
After.
</code></pre>
Before.
<# This be a comment. #>
After.
#comments
prevail over #inline-code
:
`Before. <# This be a comment. #> After.`
<code>Before. After.</code>
Before. After.
#literals
to make #inline-code
prevail over #comments
:
` <`Before. <# This be a comment. #> After.`> `
<code>Before. <# This be a comment. #> After.</code>
Before. <# This be a comment. #> After.
#divisions
ExtensibleFenceReplacement: #divisions
- queue_position: AFTER #comments
- syntax_type: BLOCK
- extensible_delimiter: ||
- attribute_specifications: EMPTY
- content_replacements:
#divisions
#prepend-newline
- tag_name: div
||
«content»
||
||{«attribute specifications»}
«content»
||
|
may be increased arbitrarily.
«attribute specifications»
:
see CMD attribute specifications.
Produces a division:
<div«attribute sequence»>
«content»
</div>
||{#test-div-id .test-div-class}
This be a division.
||
<div id="test-div-id" class="test-div-class">
This be a division.
</div>
||||{.outer}
||{.inner}
This be a division.
||
||||
<div class="outer">
<div class="inner">
This be a division.
</div>
</div>
#blockquotes
ExtensibleFenceReplacement: #blockquotes
- queue_position: AFTER #divisions
- syntax_type: BLOCK
- extensible_delimiter: ""
- attribute_specifications: EMPTY
- content_replacements:
#blockquotes
#prepend-newline
- tag_name: blockquote
""
«content»
""
""{«attribute specifications»}
«content»
""
"
may be increased arbitrarily.
«attribute specifications»
:
see CMD attribute specifications.
Produces a blockquote:
<blockquote«attribute sequence»>
«content»
</blockquote>
""{#test-blockquote-id .test-blockquote-class}
This be a blockquote.
""
<blockquote id="test-blockquote-id" class="test-blockquote-class">
This be a blockquote.
</blockquote>
#unordered-lists
ExtensibleFenceReplacement: #unordered-lists
- queue_position: AFTER #blockquotes
- syntax_type: BLOCK
- extensible_delimiter: ==
- attribute_specifications: EMPTY
- content_replacements:
#unordered-lists
#unordered-list-items
#prepend-newline
- tag_name: ul
==
- «item»
«...»
==
=={«attribute specifications»}
-{«attribute specifications»} «item»
«...»
==
=
may be increased arbitrarily.
-
, +
, or *
,
see #unordered-list-items
.
«attribute specifications»
:
see CMD attribute specifications.
Produces an unordered list:
<ul«attribute sequence»>
<li>
«item»
</li>
«...»
</ul>
======
* A
===={style="background: yellow"}
- A1
- A2
====
- B
====
+{style="color: purple"} B1
- B2
====
======
#ordered-lists
ExtensibleFenceReplacement: #ordered-lists
- queue_position: AFTER #unordered-lists
- syntax_type: BLOCK
- extensible_delimiter: ++
- attribute_specifications: EMPTY
- content_replacements:
#ordered-lists
#ordered-list-items
#prepend-newline
- tag_name: ol
++
1. «item»
«...»
++
++{«attribute specifications»}
1.{«attribute specifications»} «item»
«...»
++
+
may be increased arbitrarily.
#ordered-list-items
.
«attribute specifications»
:
see CMD attribute specifications.
Produces an ordered list:
<ol«attribute sequence»>
<li>
«item»
</li>
«...»
</ol>
++
1. A
2. B
3. C
0. D
99999999. E
++
++++
1. This shall be respected.
2. This shall be read aloud if:
++{type="a"}
1. I say so;
2. I think so; or
3.{style="color: purple"} Pigs fly.
++
++++
++{start=0}
0. Nil
1. One
++
#tables
ExtensibleFenceReplacement: #tables
- queue_position: AFTER #ordered-lists
- syntax_type: BLOCK
- extensible_delimiter: ''
- attribute_specifications: EMPTY
- content_replacements:
#tables
#table-head
#table-body
#table-foot
#table-rows
#prepend-newline
- tag_name: table
''
|^
//
; «item»
, «item»
«...»
«...»
|:
«...»
|_
«...»
''
''
//
; «item»
, «item»
«...»
«...»
''
''{«attribute specifications»}
|^{«attribute specifications»}
//{«attribute specifications»}
;{«attribute specifications»} «item»
,{«attribute specifications»} «item»
«...»
«...»
|:{«attribute specifications»}
«...»
|_{«attribute specifications»}
«...»
''
'
may be increased arbitrarily.
|^
for table head, see #table-head
.
|:
for table body, see #table-body
.
|:
for table foot, see #table-foot
.
;
for table headers, see #table-headers
.
,
for table data, see #table-data
.
«attribute specifications»
:
see CMD attribute specifications.
Produces a table:
<table«attribute sequence»>
«...»
</table>
''
//
; A
, 1
//
; B
, 2
''
<table>
<tr>
<th>A</th>
<td>1</td>
</tr>
<tr>
<th>B</th>
<td>2</td>
</tr>
</table>
A | 1 |
---|---|
B | 2 |
''
|^
//
; A
; B
|:
//
, 1
, 2
//
, First
, Second
''
<table>
<thead>
<tr>
<th>A</th>
<th>B</th>
</tr>
</thead>
<tbody>
<tr>
<td>1</td>
<td>2</td>
</tr>
<tr>
<td>First</td>
<td>Second</td>
</tr>
</tbody>
</table>
A | B |
---|---|
1 | 2 |
First | Second |
''
//
,{c3} 3*1
, 14
//
, 21
, 22
, 23
, 24
//
,{rowspan=2 colspan="2"} 2*2
, 33
,{r2} 2*1
//
, 43
''
3*1 | 14 | ||
21 | 22 | 23 | 24 |
2*2 | 33 | 2*1 | |
43 |
#headings
HeadingReplacement: #headings
- queue_position: AFTER #tables
- attribute_specifications: EMPTY
# «content»
#{«attribute specifications»} «content»
#
may be between 1 and 6.
«content»
may be optionally closed by hashes.
«content»
,
indent the continuation more than the leading hashes.
«attribute specifications»
:
see CMD attribute specifications.
Produces a heading:
<h«hash count»>«content»</h«hash count»>
#
##
###
####
#####
######
<h1></h1>
<h2></h2>
<h3></h3>
<h4></h4>
<h5></h5>
<h6></h6>
## OK
#lacks-whitespace
<h2>OK</h2>
#lacks-whitespace
## Closed ##
## Fewer closing hashes is OK #
## More closing hashes is OK ###
<h2>Closed</h2>
<h2>Fewer closing hashes is OK</h2>
<h2>More closing hashes is OK</h2>
##{.interesting-heading-class}
This heading is so long, I have used continuation.
Second continuation line.
This line is not a continuation due to insufficient indentation.
<h2 class="interesting-heading-class">
This heading is so long, I have used continuation.
Second continuation line.</h2>
This line is not a continuation due to insufficient indentation.
#paragraphs
ExtensibleFenceReplacement: #paragraphs
- queue_position: AFTER #headings
- syntax_type: BLOCK
- extensible_delimiter: --
- attribute_specifications: EMPTY
- prohibited_content: BLOCKS
- content_replacements:
#prepend-newline
- tag_name: p
--
«content»
--
--{«attribute specifications»}
«content»
--
-
may be increased arbitrarily.
«attribute specifications»
:
see CMD attribute specifications.
Produces a paragraph:
<p«attribute sequence»>
«content»
</p>
--
The quick brown fox etc. etc.
--
<p>
The quick brown fox etc. etc.
</p>
The quick brown fox etc. etc.
----
Before.
--
Not a nested paragraph.
--
After.
----
<p>
Before.
--
Not a nested paragraph.
--
After.
</p>
Before. -- Not a nested paragraph. -- After.
#inline-code
ExtensibleFenceReplacement: #inline-code
- queue_position: AFTER #paragraphs
- syntax_type: INLINE
- allowed_flags:
u=KEEP_HTML_UNESCAPED
i=KEEP_INDENTED
w=REDUCE_WHITESPACE
- extensible_delimiter: `
- attribute_specifications: EMPTY
- prohibited_content: ANCHORED_BLOCKS
- content_replacements:
#escape-html
#de-indent
#trim-whitespace
#reduce-whitespace
#placeholder-protect
- tag_name: code
` «content» `
`{«attribute specifications»} «content» `
«flags»` «content» `
«flags»`{«attribute specifications»} «content» `
«flags»
:
u
: keep HTML unescaped (do not apply #escape-html
)
i
: keep indented (do not apply #de-indent
)
w
: reduce whitespace (apply #reduce-whitespace
)
`
may be increased arbitrarily.
«attribute specifications»
:
see CMD attribute specifications.
Produces inline code:
<code«attribute sequence»>«content»</code>
`<br>`
<code><br></code>
<br>
``A `backticked` word.``
<code>A `backticked` word.</code>
A `backticked` word.
#literals
with flag u
to inject HTML:
`Some u<`<b>bold</b>`> code`
<code>Some <b>bold</b> code</code>
Some bold code
#boilerplate
RegexDictionaryReplacement: #boilerplate
- queue_position: AFTER #inline-code
* \A -->
<!DOCTYPE html>
<html lang="%lang">
<head>
<meta charset="utf-8">
%head-elements-before-viewport
<meta name="viewport" content="%viewport-content">
%head-elements-after-viewport
<title>%title</title>
<style>
%styles
</style>
</head>
<body>\n
* \Z -->
</body>
</html>\n
Wraps content in the HTML5 boilerplate:
<!DOCTYPE html>
through <body>
at the start
</body></html>
at the end
The default boilerplate properties
%lang
,
%head-elements-before-viewport
,
%viewport-content
,
%head-elements-after-viewport
,
%title
, and
%styles
are set in #boilerplate-properties
.
#boilerplate-properties
OrdinaryDictionaryReplacement: #boilerplate-properties
- queue_position: AFTER #boilerplate
* %lang --> en
* %head-elements-before-viewport -->
* %viewport-content --> width=device-width, initial-scale=1
* %head-elements-after-viewport -->
* %title --> Title
* %styles -->
Makes replacements for the default boilerplate properties:
Property | Default value |
---|---|
%lang |
en |
%head-elements-before-viewport |
(empty) |
%viewport-content |
width=device-width, initial-scale=1 |
%head-elements-after-viewport |
(empty) |
%title |
Title |
%styles |
(empty) |
# %title
This document hath `lang` equal to <code>%lang</code>.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>Title</title>
</head>
<body>
<h1>Title</h1>
This document hath <code>lang</code> equal to <code>en</code>.
</body>
</html>
OrdinaryDictionaryReplacement: #.boilerplate-properties-override
- queue_position: BEFORE #boilerplate-properties
* %lang --> en-AU
* %head-elements-before-viewport --> <meta name="author" content="Me">
* %title --> Overridden title
%%%
# %title
This document hath `lang` equal to <code>%lang</code>.
<!DOCTYPE html>
<html lang="en-AU">
<head>
<meta charset="utf-8">
<meta name="author" content="Me">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>Overridden title</title>
</head>
<body>
<h1>Overridden title</h1>
This document hath <code>lang</code> equal to <code>en-AU</code>.
</body>
</html>
#cmd-properties
OrdinaryDictionaryReplacement: #cmd-properties
- queue_position: AFTER #boilerplate-properties
* %cmd-version --> CMD_VERSION
* %cmd-name --> CMD_NAME
* %cmd-basename --> CMD_BASENAME
* %clean-url --> CLEAN_URL
- concluding_replacements:
#placeholder-protect
Makes replacements for CMD properties:
Property | Description |
---|---|
%cmd-version |
__version__ in _version.py
(currently 5.0.1 ) |
%cmd-name |
CMD file name, relative to working directory, without extension |
%cmd-basename |
CMD file name, without path, without extension |
%clean-url |
%cmd-name ,
with %cmd-basename removed if it equals index |
#boilerplate-protect
RegexDictionaryReplacement: #boilerplate-protect
- queue_position: AFTER #cmd-properties
* <style>[\s]*?</style>[\s]* -->
* <style>[\s\S]*?</style> --> \g<0>
* <head>[\s\S]*?</head> --> \g<0>
- concluding_replacements:
#reduce-whitespace
#placeholder-protect
Protects boilerplate elements:
<style>
element
<style>
element
<head>
element
#backslash-escapes
OrdinaryDictionaryReplacement: #backslash-escapes
- queue_position: AFTER #boilerplate-protect
* \\ --> \
* \" --> "
* \# --> #
* \& --> &
* \' --> '
* \( --> (
* \) --> )
* \* --> *
* \< --> <
* \> --> >
* \[ --> [
* \] --> ]
* \_ --> _
* \{ --> {
* \| --> |
* \} --> }
* "\ " --> " "
* \t --> " "
- concluding_replacements:
#placeholder-protect
Applies backslash escapes:
Escaped | Unescaped |
---|---|
\\ |
\ |
\" |
" |
\# |
# |
\& |
& |
\' |
' |
\( |
( |
\) |
) |
\* |
* |
\< |
< |
\> |
> |
\[ |
[ |
\] |
] |
\_ |
_ |
\{ |
{ |
\| |
| |
\} |
} |
\ |
(space) |
\t |
(tab) |
#backslash-continuations
RegexDictionaryReplacement: #backslash-continuations
- queue_position: AFTER #backslash-escapes
* \\ \n [^\S\n]* -->
Applies backslash continuation.
#reference-definitions
ReferenceDefinitionReplacement: #reference-definitions
- queue_position: AFTER #backslash-continuations
- attribute_specifications: EMPTY
[«label»]: «uri»
[«label»]: <«uri»>
[«label»]: «...» "«title»"
[«label»]: «...» '«title»'
[«label»]{«attribute specifications»}: «...»
«uri»
or «title»
,
indent the continuation more than the leading square bracket.
«attribute specifications»
:
see CMD attribute specifications.
Defines a reference, to be used by
#referenced-images
or #referenced-links
.
#specified-images
SpecifiedImageReplacement: #specified-images
- queue_position: AFTER #reference-definitions
- attribute_specifications: EMPTY
- prohibited_content: BLOCKS




![«alt text»]{«attribute specifications»}(«...»)
«attribute specifications»
:
see CMD attribute specifications.
Produces an image:
<img«attribute sequence»>
Here, «attribute sequence»
is the sequence of attributes
built from
«alt text»
«src»
«title»
«attribute specifications»
parsed in that order.
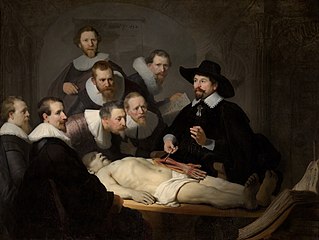
<img alt="Rembrandt painting: The Anatomy Lesson of Dr Nicolaes Tulp." src="rembrandt-anatomy.jpg">
![Rembrandt painting: The Anatomy Lesson of Dr Nicolaes Tulp.]{w=120}(rembrandt-anatomy.jpg)
<img alt="Rembrandt painting: The Anatomy Lesson of Dr Nicolaes Tulp." src="rembrandt-anatomy.jpg" width="120">
 Conway-Markdown is dumb.
<img alt="" src="/favicon-16x16.png"> Conway-Markdown is dumb.
Conway-Markdown is dumb.
#referenced-images
ReferencedImageReplacement: #referenced-images
- queue_position: AFTER #specified-images
- attribute_specifications: EMPTY
- prohibited_content: BLOCKS
![«alt text»][«label»]
![«alt text»]
![«alt text»]{«attribute specifications»}[«label»]
![«alt text»]{«attribute specifications»}
«label»
: must correspond to a defined
reference definition, up to case.
If «label»
is omitted,
«alt text»
is used in its stead.
«attribute specifications»
:
see CMD attribute specifications.
Produces an image:
<img«attribute sequence»>
Here, «attribute sequence»
is the sequence of attributes
built from
«alt text»
«src»
equal to «uri»
of the reference definition
defined for «label»
«title»
of the reference definition
defined for «label»
«attribute specifications»
of the reference definition
defined for «label»
«attribute specifications»
parsed in that order.
[moses]: rembrandt-moses.jpg
![Rembrandt painting: Moses Breaking the Tablets of the Law.][moses]
<img alt="Rembrandt painting: Moses Breaking the Tablets of the Law." src="rembrandt-moses.jpg">
«alt text»
for «label»
:
[Conway-Markdown logo.]: /favicon-32x32.png "Conway-Markdown is dumb."
![Conway-Markdown logo.]
<img alt="Conway-Markdown logo." src="/favicon-32x32.png" title="Conway-Markdown is dumb.">
«label»
is case-insensitive:
[image-label-case]: insensitive.png
[Hooray.][image-label-case]
[Hooray.][image-label-CASE]
[Hooray.][ImAGe-laBEl-CAsE]
<a href="insensitive.png">Hooray.</a>
<a href="insensitive.png">Hooray.</a>
<a href="insensitive.png">Hooray.</a>
[image-label]{.class1}: file1.png
[image-label]{.class2}: file2.png
[Second definition wins.][image-label]
<a href="file2.png" class="class2">Second definition wins.</a>
#explicit-links
ExplicitLinkReplacement: #explicit-links
- queue_position: AFTER #referenced-images
- allowed_flags:
b=ANGLE_BRACKET_WRAP
s=SUPPRESS_SCHEME
- attribute_specifications: EMPTY
- content_replacements:
#suppress-scheme
- concluding_replacements:
#angle-bracket-wrap
<«uri»>
<{«attribute specifications»} «uri»>
«flags»<«uri»>
«flags»<{«attribute specifications»} «uri»>
«flags»
:
b
: wrap in angle brackets (apply #angle-bracket-wrap
)
s
: suppress scheme (apply #suppress-scheme
)
«attribute specifications»
:
see CMD attribute specifications.
Produces a link:
<a«attribute sequence»>«uri»</a>
Or:
<<a«attribute sequence»>«uri»</a>>
Here, «attribute sequence»
is the sequence of attributes
built from
«uri»
as href
«attribute specifications»
parsed in that order.
<https://example.com>
<a href="https://example.com">https://example.com</a>
b<https://example.com>
<<a href="https://example.com">https://example.com</a>>
s<https://example.com>
<a href="https://example.com">example.com</a>
bs<https://example.com>
<<a href="https://example.com">example.com</a>>
s<mailto:mail@example.com>
<a href="mailto:mail@example.com">mail@example.com</a>
#specified-links
SpecifiedLinkReplacement: #specified-links
- queue_position: AFTER #explicit-links
- attribute_specifications: EMPTY
- prohibited_content: BLOCKS
[«link text»](«href»)
[«link text»](<«href»>)
[«link text»](«...» "«title»")
[«link text»](«...» '«title»')
[«link text»]{«attribute specifications»}(«...»)
«attribute specifications»
:
see CMD attribute specifications.
Produces a link:
<a«attribute sequence»>«link text»</a>
Here, «attribute sequence»
is the sequence of attributes
built from
«href»
«title»
«attribute specifications»
parsed in that order.
[Wikipedia](https://en.wikipedia.org/wiki/Main_Page)
<a href="https://en.wikipedia.org/wiki/Main_Page">Wikipedia</a>
[Wikipedia](
https://en.wikipedia.org/wiki/Main_Page
"Wikipedia, the free encyclopedia"
)
<a href="https://en.wikipedia.org/wiki/Main_Page" title="Wikipedia, the free encyclopedia">Wikipedia</a>
href
[Beware]{href=https://example.com/evil/}(https://example.com/good/)
<a href="https://example.com/evil/">Beware</a>
#referenced-links
ReferencedLinkReplacement: #referenced-links
- queue_position: AFTER #specified-links
- attribute_specifications: EMPTY
- prohibited_content: BLOCKS
[«link text»][«label»]
[«link text»]
[«link text»]{«attribute specifications»}[«label»]
[«link text»]{«attribute specifications»}
«label»
: must correspond to a defined
reference definition, up to case.
If «label»
is omitted,
«link text»
is used in its stead.
«attribute specifications»
:
see CMD attribute specifications.
Produces a link:
<a«attribute sequence»>«link text»</a>
Here, «attribute sequence»
is the sequence of attributes
built from
«href»
equal to «uri»
of the reference definition
defined for «label»
«title»
of the reference definition
defined for «label»
«attribute specifications»
of the reference definition
defined for «label»
«attribute specifications»
parsed in that order.
[wiki]: https://en.wikipedia.org/wiki/Main_Page
[Wikipedia's main page.][wiki]
<a href="https://en.wikipedia.org/wiki/Main_Page">Wikipedia's main page.</a>
«link text»
for «label»
:
[Wikipedia]: https://en.wikipedia.org/wiki/Main_Page
[Wikipedia]
<a href="https://en.wikipedia.org/wiki/Main_Page">Wikipedia</a>
«label»
is case-insensitive:
[link-label-case]: https://example.com
[Hooray.][link-label-case]
[Hooray.][link-label-CASE]
[Hooray.][LiNK-laBEl-CAsE]
<a href="https://example.com">Hooray.</a>
<a href="https://example.com">Hooray.</a>
<a href="https://example.com">Hooray.</a>
[link-label]{.class1}: https://example.com/1
[link-label]{.class2}: https://example.com/2
[Second definition wins.][link-label]
<a href="https://example.com/2" class="class2">Second definition wins.</a>
#inline-semantics
InlineAssortedDelimitersReplacement: #inline-semantics
- queue_position: AFTER #referenced-links
- delimiter_conversion:
__=b
_=i
**=strong
*=em
''=cite
""=q
- attribute_specifications: EMPTY
- prohibited_content: BLOCKS
__«b content»__
_«i content»_
**«strong content»**
*«em content»*
''«cite content»''
""«q content»""
«...»{«attribute specifications»} «content»«...»
|«...»«content»«...»
|«...»{«attribute specifications»} «content»«...»
«attribute specifications»
:
see CMD attribute specifications.
</
(the beginning of a closing tag).
|
.
Produces an inline semantic:
<b«attribute sequence»>«b content»</b>
<i«attribute sequence»>«i content»</i>
<strong«attribute sequence»>«strong content»</strong>
<em«attribute sequence»>«em content»</em>
<cite«attribute sequence»>«cite content»</cite>
<q«attribute sequence»>«q content»</q>
In HTML5, <b>
and <i>
are not deprecated,
see W3C: Using <b>
and <i>
elements.
<b>
:
Meals are served with __rice__ or __pasta__.
Meals are served with <b>rice</b> or <b>pasta</b>.
<i>
:
Write out _{lang=la} Romani ite domum_ 100 times.
Write out <i lang="la">Romani ite domum</i> 100 times.
I _{.translator-supplied} am_ the LORD.
I <i class="translator-supplied">am</i> the LORD.
_Screw 'em._
<i>Screw 'em.</i>
<strong>
:
**{lang=de} Achtung!**
<strong lang="de">Achtung!</strong>
<em>
:
I don't know *who* took it.
I don't know <em>who</em> took it.
<cite>
:
''Nineteen Eighty-Four'' is already upon us.
<cite>Nineteen Eighty-Four</cite> is already upon us.
<q>
:
""It wasn't me.""
<q>It wasn't me.</q>
It wasn't me.
Pattern | CMD | HTML |
---|---|---|
1-1 | *foo* |
<em>foo</em> |
2-2 | **foo** |
<strong>foo</strong> |
3-3 (12-21) | ***foo*** |
<em><strong>foo</strong></em> |
12-3 (12-21) | *foo **bar*** |
<em>foo <strong>bar</strong></em> |
3-21 (12-21) | ***foo** bar* |
<em><strong>foo</strong> bar</em> |
2|1-3 (21-12) | **|*foo*** |
<strong><em>foo</em></strong> |
21-3 (21-12) | **foo *bar*** |
<strong>foo <em>bar</em></strong> |
3-12 (21-12) | ***foo* bar** |
<strong><em>foo</em> bar</strong> |
4-4 (22-22) | ****foo**** |
<strong><strong>foo</strong></strong> |
1|2|1-4 (121-121) | *|**|*foo**** |
<em><strong><em>foo</em></strong></em> |
#escape-idle-html
RegexDictionaryReplacement: #escape-idle-html
- queue_position: AFTER #inline-semantics
* [&]
(?!
(?:
[a-zA-Z]{1,31}
|
[#] (?: [0-9]{1,7} | [xX] [0-9a-fA-F]{1,6} )
)
[;]
)
--> &
* [<] (?= [\s] ) --> <
Escapes idle HTML:
&
.
<
#whitespace
ReplacementSequence: #whitespace
- queue_position: AFTER #escape-idle-html
- replacements:
#reduce-whitespace
Reduces whitespace. See #reduce-whitespace
.
#placeholder-unprotect
PlaceholderUnprotectionReplacement: #placeholder-unprotect
- queue_position: AFTER #whitespace
Unprotects all placeholder strings.
#placeholder-protect
PlaceholderProtectionReplacement: #placeholder-protect
Protects a string with a placeholder.
#de-indent
DeIndentationReplacement: #de-indent
- negative_flag: KEEP_INDENTED
Removes the longest common indentation in a string.
#escape-html
OrdinaryDictionaryReplacement: #escape-html
- negative_flag: KEEP_HTML_UNESCAPED
* & --> &
* < --> <
* > --> >
Escapes &
, <
, and >
as their respective HTML ampersand entities.
#trim-whitespace
RegexDictionaryReplacement: #trim-whitespace
* \A [\s]* -->
* [\s]* \Z -->
Removes whitespace at the very start and very end of the string.
#reduce-whitespace
RegexDictionaryReplacement: #reduce-whitespace
- positive_flag: REDUCE_WHITESPACE
* ^ [^\S\n]+ -->
* [^\S\n]+ $ -->
* [\s]+ (?= <br> ) -->
* [\n]+ --> \n
<br>
.
#code-tag-wrap
RegexDictionaryReplacement: #code-tag-wrap
* \A --> <code>
* \Z --> </code>
Wraps a string in opening and closing code
tags.
#prepend-newline
RegexDictionaryReplacement: #prepend-newline
* \A --> \n
Adds a newline at the very start of a string.
#unordered-list-items
PartitioningReplacement: #unordered-list-items
- starting_pattern: [-+*]
- attribute_specifications: EMPTY
- content_replacements:
#prepend-newline
- ending_pattern: [-+*]
- tag_name: li
Partitions content into list items based on leading occurrences of
-
, +
, or *
.
#ordered-list-items
PartitioningReplacement: #ordered-list-items
- starting_pattern: [0-9]+ [.]
- attribute_specifications: EMPTY
- content_replacements:
#prepend-newline
- ending_pattern: [0-9]+ [.]
- tag_name: li
Partitions content into list items based on leading occurrences of a run of digits followed by a full stop.
#mark-table-headers-for-preceding-table-data
RegexDictionaryReplacement: #mark-table-headers-for-preceding-table-data
* \A --> ;{}
# Replaces `<th«attributes_sequence»>` with `;{}<th«attributes_sequence»>`,
# so that #table-data will know to stop before it.
Replaces <th«attributes_sequence»>
with ;{}<th«attributes_sequence»>
so that #table-data
will know to stop before it.
#table-headers
PartitioningReplacement: #table-headers
- starting_pattern: [;]
- attribute_specifications: EMPTY
- content_replacements:
#trim-whitespace
- ending_pattern: [;,]
- tag_name: th
- concluding_replacements:
#mark-table-headers-for-preceding-table-data
Partitions content into table headers
based on leading occurrences of ;
up to the next leading ;
or ,
.
#table-data
PartitioningReplacement: #table-data
- starting_pattern: [,]
- attribute_specifications: EMPTY
- content_replacements:
#trim-whitespace
- ending_pattern: [;,]
- tag_name: td
Partitions content into table data
based on leading occurrences of ,
up to the next leading ;
or ,
.
#unmark-table-headers-for-preceding-table-data
RegexDictionaryReplacement: #unmark-table-headers-for-preceding-table-data
* ^ [;] \{ \} <th (?P<bracket_or_placeholder_marker> [>\uF8FF] )
-->
<th\g<bracket_or_placeholder_marker>
# Replaces `;{}<th«attributes_sequence»>` with `<th«attributes_sequence»>`
# so that #mark-table-headers-for-preceding-table-data is undone.
Replaces ;{}<th«attributes_sequence»>
with <th«attributes_sequence»>
so that #mark-table-headers-for-preceding-table-data
is undone.
#table-rows
PartitioningReplacement: #table-rows
- starting_pattern: [/]{2}
- attribute_specifications: EMPTY
- ending_pattern: [/]{2}
- content_replacements:
#table-headers
#table-data
#unmark-table-headers-for-preceding-table-data
#prepend-newline
- tag_name: tr
Partitions content into table rows
based on leading occurrences of //
.
#table-head
PartitioningReplacement: #table-head
- starting_pattern: [|][\^]
- attribute_specifications: EMPTY
- ending_pattern: [|][:_]
- content_replacements:
#table-rows
#prepend-newline
- tag_name: thead
Partitions content into table heads
based on leading occurrences of |^
up to the next leading |:
or |_
.
#table-body
PartitioningReplacement: #table-body
- starting_pattern: [|][:]
- attribute_specifications: EMPTY
- ending_pattern: [|][_]
- content_replacements:
#table-rows
#prepend-newline
- tag_name: tbody
Partitions content into table bodies
based on leading occurrences of |:
up to the next leading |_
.
#table-foot
PartitioningReplacement: #table-foot
- starting_pattern: [|][_]
- attribute_specifications: EMPTY
- content_replacements:
#table-rows
#prepend-newline
- tag_name: tfoot
Partitions content into table feet
based on leading occurrences of |_
.
#suppress-scheme
RegexDictionaryReplacement: #suppress-scheme
- positive_flag: SUPPRESS_SCHEME
* \A [\S]+ [:] (?: [/]{2} )? -->
Suppresses the scheme (including the colon and possibly two slashes) of a URI.
#angle-bracket-wrap
RegexDictionaryReplacement: #angle-bracket-wrap
- positive_flag: ANGLE_BRACKET_WRAP
* \A --> <
* \Z --> >
- concluding_replacements:
#placeholder-protect
Wraps a string in angle brackets.
This section gives the syntax for writing user-defined CMD replacement rules,
which go before the «delimiter»
when authoring CMD files.
In CMD replacement rule syntax, a line must be one of the following:
# «comment»
Is ignored.
< «included_file_name»
Includes the content of «included_file_name»
as CMD replacement rules.
«included_file_name»
begins with a slash,
it is parsed relative to the working directory.
«included_file_name»
does not begin with a slash,
it is parsed relative to the file in which the inclusion is invoked.
«included_file_name»
to have the extension .cmdr
.
«ClassName»: #«id»
Begins the definition of a replacement rule
with class «ClassName»
.
«ClassName»
must be one of
ReplacementSequence
,
PlaceholderMarkerReplacement
,
PlaceholderProtectionReplacement
,
PlaceholderUnprotectionReplacement
,
DeIndentationReplacement
,
OrdinaryDictionaryReplacement
,
RegexDictionaryReplacement
,
FixedDelimitersReplacement
,
ExtensibleFenceReplacement
,
PartitioningReplacement
,
InlineAssortedDelimitersReplacement
,
HeadingReplacement
,
ReferenceDefinitionReplacement
,
SpecifiedImageReplacement
,
ReferencedImageReplacement
,
ExplicitLinkReplacement
,
SpecifiedLinkReplacement
,
or
ReferencedLinkReplacement
.
«id»
may only contain
lower-case letters, digits, hyphens, and full stops.
The convention is for user-defined replacement rules
to have «id»
beginning with a full stop
(whereas the standard rules
have «id»
beginning with a letter or digit).
- «name»: «value»
Declares an attribute for the replacement that is currently being defined.
«name»
must be one of the valid attribute names
for the replacement that is currently being defined.
«value»
must be a suitable attribute value
for that attribute name.
* «pattern» --> «substitute»
Declares a substitution for the replacement that is currently being defined
(which must be an OrdinaryDictionaryReplacement
or a RegexDictionaryReplacement
).
-->
may be arbitrarily increased should «pattern»
contain
a run of hyphens followed by a closing angle-bracket.
ReplacementSequence
ReplacementSequence: #«id»
- queue_position: (def) NONE | ROOT | BEFORE #«id» | AFTER #«id»
- replacements: (def) NONE | #«id» [...]
queue_position
:
position in the replacement queue
replacements
:
sequence of replacements to be applied
Defines a replacement rule that applies a sequence of replacement rules.
PlaceholderMarkerReplacement
PlaceholderMarkerReplacement: #«id»
- queue_position: (def) NONE | ROOT | BEFORE #«id» | AFTER #«id»
queue_position
:
position in the replacement queue
Defines a rule for replacing the placeholder marker with a placeholder.
PlaceholderProtectionReplacement
PlaceholderProtectionReplacement: #«id»
- queue_position: (def) NONE | ROOT | BEFORE #«id» | AFTER #«id»
queue_position
:
position in the replacement queue
Defines a replacement rule for protecting strings with a placeholder.
PlaceholderUnprotectionReplacement
PlaceholderUnprotectionReplacement: #«id»
- queue_position: (def) NONE | ROOT | BEFORE #«id» | AFTER #«id»
queue_position
:
position in the replacement queue
Defines a replacement rule for restoring placeholders to their strings.
DeIndentationReplacement
DeIndentationReplacement: #«id»
- queue_position: (def) NONE | ROOT | BEFORE #«id» | AFTER #«id»
- positive_flag: (def) NONE | «FLAG_NAME»
- negative_flag: (def) NONE | «FLAG_NAME»
queue_position
:
position in the replacement queue
positive_flag
:
name of the flag that must be enabled for the replacement to be applied
negative_flag
:
name of the flag that must not be enabled for the replacement to be applied
Defines a replacement rule for de-indentation.
OrdinaryDictionaryReplacement
OrdinaryDictionaryReplacement: #«id»
- queue_position: (def) NONE | ROOT | BEFORE #«id» | AFTER #«id»
- positive_flag: (def) NONE | «FLAG_NAME»
- negative_flag: (def) NONE | «FLAG_NAME»
- apply_mode: (def) SIMULTANEOUS | SEQUENTIAL
* "«pattern»" | '«pattern»' | «pattern»
-->
CMD_VERSION | CMD_NAME | CMD_BASENAME | CLEAN_URL |
"«substitute»" | '«substitute»' | «substitute»
[...]
- concluding_replacements: (def) NONE | #«id» [...]
queue_position
:
position in the replacement queue
positive_flag
:
name of the flag that must be enabled for the replacement to be applied
negative_flag
:
name of the flag that must not be enabled for the replacement to be applied
apply_mode
:
whether the substitutions are to be applied simultaneously or sequentially
Keyword | Description |
---|---|
CMD_VERSION |
__version__ in _version.py
(currently 5.0.1 ) |
CMD_NAME |
CMD file name, relative to working directory, without extension |
CMD_BASENAME |
CMD file name, without path, without extension |
CLEAN_URL |
CMD_NAME , with CMD_BASENAME removed if it equals index |
concluding_replacements
:
sequence of replacements to be applied after substitution
Defines a replacement rule for a dictionary of ordinary substitutions.
RegexDictionaryReplacement
RegexDictionaryReplacement: #«id»
- queue_position: (def) NONE | ROOT | BEFORE #«id» | AFTER #«id»
- positive_flag: (def) NONE | «FLAG_NAME»
- negative_flag: (def) NONE | «FLAG_NAME»
* "«pattern»" | '«pattern»' | «pattern»
-->
CMD_VERSION | CMD_NAME | CMD_BASENAME | CLEAN_URL |
"«substitute»" | '«substitute»' | «substitute»
[...]
- concluding_replacements: (def) NONE | #«id» [...]
queue_position
:
position in the replacement queue
positive_flag
:
name of the flag that must be enabled for the replacement to be applied
negative_flag
:
name of the flag that must not be enabled for the replacement to be applied
apply_mode
:
whether the substitutions are to be applied simultaneously or sequentially
Keyword | Description |
---|---|
CMD_VERSION |
__version__ in _version.py
(currently 5.0.1 ) |
CMD_NAME |
CMD file name, relative to working directory, without extension |
CMD_BASENAME |
CMD file name, without path, without extension |
CLEAN_URL |
CMD_NAME , with CMD_BASENAME removed if it equals index |
flags=re.ASCII | re.MULTILINE | re.VERBOSE
.
concluding_replacements
:
sequence of replacements to be applied after substitution
Defines a replacement rule for a dictionary of regex substitutions.
FixedDelimitersReplacement
FixedDelimitersReplacement: #«id»
- queue_position: (def) NONE | ROOT | BEFORE #«id» | AFTER #«id»
- syntax_type: BLOCK | INLINE (mandatory)
- allowed_flags: (def) NONE | «letter»=«FLAG_NAME» [...]
- opening_delimiter: «string» (mandatory)
- attribute_specifications: (def) NONE | EMPTY | «string»
- prohibited_content: (def) NONE | BLOCKS | ANCHORED_BLOCKS
- content_replacements: (def) NONE | #«id» [...]
- closing_delimiter: «string» (mandatory)
- tag_name: (def) NONE | «name»
- concluding_replacements: (def) NONE | #«id» [...]
queue_position
:
position in the replacement queue
syntax_type
:
whether the syntax type is block (delimiters must be on their own lines)
or inline (delimiters may be anywhere)
allowed_flags
:
flag letters and corresponding flag names
opening_delimiter
:
the opening delimiter
attribute_specifications
:
whether, after the opening delimiter,
there may be CMD attribute specifications supplied,
and if so, what the default specification is
prohibited_content
:
whether block tags or anchored block tags are prohibited
in the content between the opening and closing delimiters
content_replacements
:
sequence of replacements to be applied
to the content between the opening and closing delimiters
closing_delimiter
:
the closing delimiter
tag_name
:
the tag name of the element to be constructed
concluding_replacements
:
sequence of replacements to be applied after construction of the element
Defines a fixed-delimiters replacement rule.
(None)
ExtensibleFenceReplacement
ExtensibleFenceReplacement: #«id»
- queue_position: (def) NONE | ROOT | BEFORE #«id» | AFTER #«id»
- syntax_type: BLOCK | INLINE (mandatory)
- allowed_flags: (def) NONE | «letter»=«FLAG_NAME» [...]
- prologue_delimiter: (def) NONE | «string»
- extensible_delimiter: «character_repeated» (mandatory)
- attribute_specifications: (def) NONE | EMPTY | «string»
- prohibited_content: (def) NONE | BLOCKS | ANCHORED_BLOCKS
- content_replacements: (def) NONE | #«id» [...]
- epilogue_delimiter: (def) NONE | «string»
- tag_name: (def) NONE | «name»
- concluding_replacements: (def) NONE | #«id» [...]
queue_position
:
position in the replacement queue
syntax_type
:
whether the syntax type is block (delimiters must be on their own lines)
or inline (delimiters may be anywhere)
allowed_flags
:
flag letters and corresponding flag names
prologue_delimiter
:
the delimiter that must appear before the opening extensible delimiter
extensible_delimiter
:
the opening and closing extensible delimiter (a character repeated)
attribute_specifications
:
whether, after the opening extensible delimiter,
there may be CMD attribute specifications supplied,
and if so, what the default specification is
prohibited_content
:
whether block tags or anchored block tags are prohibited
in the content between the opening and closing extensible delimiters
content_replacements
:
sequence of replacements to be applied
to the content between the opening and closing extensible delimiters
epilogue_delimiter
:
the delimiter that must appear after the closing extensible delimiter
tag_name
:
the tag name of the element to be constructed
concluding_replacements
:
sequence of replacements to be applied after construction of the element
Defines a generalised extensible-fence-style replacement rule. (Inspired by the repeatable backticks of John Gruber's Markdown.)
PartitioningReplacement
PartitioningReplacement: #«id»
- queue_position: (def) NONE | ROOT | BEFORE #«id» | AFTER #«id»
- starting_pattern: «regex» (mandatory)
- attribute_specifications: (def) NONE | EMPTY | «string»
- content_replacements: (def) NONE | #«id» [...]
- ending_pattern: (def) NONE | «regex»
- tag_name: (def) NONE | «name»
- concluding_replacements: (def) NONE | #«id» [...]
queue_position
:
position in the replacement queue
starting_pattern
:
pattern that marks the start of a partition
attribute_specifications
:
whether, after the starting pattern,
there may be CMD attribute specifications supplied,
and if so, what the default specification is
content_replacements
:
sequence of replacements to be applied
to the content from the starting pattern up to the ending pattern
ending_pattern
:
pattern, not consumed, that marks the end of a partition
tag_name
:
the tag name of the element to be constructed
concluding_replacements
:
sequence of replacements to be applied after construction of the element
Defines a generalised partitioning replacement rule. (Does partitioning by consuming everything from a starting pattern up to but not including an ending pattern.)
InlineAssortedDelimitersReplacement
InlineAssortedDelimitersReplacement: #«id»
- queue_position: (def) NONE | ROOT | BEFORE #«id» | AFTER #«id»
- delimiter_conversion:
«character» | «character_doubled»=«tag_name» [...] (mandatory)
- attribute_specifications: (def) NONE | EMPTY | «string»
- prohibited_content: (def) NONE | BLOCKS | ANCHORED_BLOCKS
queue_position
:
position in the replacement queue
delimiter_conversion
:
a sequence of conversions
from single-character or double-character delimiters
to tag names
attribute_specifications
:
whether, after each starting delimiter,
there may be CMD attribute specifications supplied,
and if so, what the default specification is
prohibited_content
:
whether block tags or anchored block tags are prohibited
in the content between the opening and closing delimiters
Defines an inline assorted-delimiters replacement rule.
(A generalisation of the replacement that processes *
and **
into <em>
and <strong>
in Markdown.)
HeadingReplacement
HeadingReplacement: #«id»
- queue_position: (def) NONE | ROOT | BEFORE #«id» | AFTER #«id»
- attribute_specifications: (def) NONE | EMPTY | «string»
queue_position
:
position in the replacement queue
attribute_specifications
:
whether, after the declaring hashes,
there may be CMD attribute specifications supplied,
and if so, what the default specification is
Defines a replacement rule for headings. (A generalisation of the replacement that processes ATX headings in Markdown.)
ReferenceDefinitionReplacement
ReferenceDefinitionReplacement: #«id»
- queue_position: (def) NONE | ROOT | BEFORE #«id» | AFTER #«id»
- attribute_specifications: (def) NONE | EMPTY | «string»
queue_position
:
position in the replacement queue
attribute_specifications
:
whether, between the square-bracketed label and the declaring colon,
there may be CMD attribute specifications supplied,
and if so, what the default specification is
Defines a replacement rule for consuming reference definitions.
(A generalisation of the replacement that processes
[«label»]: «uri» "«title»"
in Markdown.)
SpecifiedImageReplacement
SpecifiedImageReplacement: #«id»
- queue_position: (def) NONE | ROOT | BEFORE #«id» | AFTER #«id»
- attribute_specifications: (def) NONE | EMPTY | «string»
- prohibited_content: (def) NONE | BLOCKS | ANCHORED_BLOCKS
queue_position
:
position in the replacement queue
attribute_specifications
:
whether, between the square-bracketed alt text
and the round-bracketed URI & title,
there may be CMD attribute specifications supplied,
and if so, what the default specification is
prohibited_content
:
whether block tags or anchored block tags are prohibited
in the square-bracketed alt text
Defines a replacement rule for specified images.
(A generalisation of the replacement that processes

in Markdown.)
ReferencedImageReplacement
ReferencedImageReplacement: #«id»
- queue_position: (def) NONE | ROOT | BEFORE #«id» | AFTER #«id»
- attribute_specifications: (def) NONE | EMPTY | «string»
- prohibited_content: (def) NONE | BLOCKS | ANCHORED_BLOCKS
queue_position
:
position in the replacement queue
attribute_specifications
:
whether, after the square-bracketed alt text,
there may be CMD attribute specifications supplied,
and if so, what the default specification is
prohibited_content
:
whether block tags or anchored block tags are prohibited
in the square-bracketed alt text and the square-bracketed label
Defines a replacement rule for referenced images.
(A generalisation of the replacement that processes
![«alt text»][«label»]
in Markdown.)
ExplicitLinkReplacement
ExplicitLinkReplacement: #«id»
- queue_position: (def) NONE | ROOT | BEFORE #«id» | AFTER #«id»
- allowed_flags: (def) NONE | «letter»=«FLAG_NAME» [...]
- attribute_specifications: (def) NONE | EMPTY | «string»
- content_replacements: (def) NONE | #«id» [...]
- concluding_replacements: (def) NONE | #«id» [...]
queue_position
:
position in the replacement queue
allowed_flags
:
flag letters and corresponding flag names
attribute_specifications
:
whether, after the opening angle bracket,
there may be CMD attribute specifications supplied,
and if so, what the default specification is
content_replacements
:
sequence of replacements to be applied
to the content between the opening and closing angle brackets
concluding_replacements
:
sequence of replacements to be applied after construction of the element
Defines a replacement rule for explicit links.
(A generalisation of the replacement that processes
<«uri»>
in Markdown.)
SpecifiedLinkReplacement
SpecifiedLinkReplacement: #«id»
- queue_position: (def) NONE | ROOT | BEFORE #«id» | AFTER #«id»
- attribute_specifications: (def) NONE | EMPTY | «string»
- prohibited_content: (def) NONE | BLOCKS | ANCHORED_BLOCKS
queue_position
:
position in the replacement queue
attribute_specifications
:
whether, between the square-bracketed link text
and the round-bracketed URI & title,
there may be CMD attribute specifications supplied,
and if so, what the default specification is
prohibited_content
:
whether block tags or anchored block tags are prohibited
in the square-bracketed link text
Defines a replacement rule for specified links.
(A generalisation of the replacement that processes
[«link text»](«uri» "«title»")
in Markdown.)
ReferencedLinkReplacement
ReferencedLinkReplacement: #«id»
- queue_position: (def) NONE | ROOT | BEFORE #«id» | AFTER #«id»
- attribute_specifications: (def) NONE | EMPTY | «string»
- prohibited_content: (def) NONE | BLOCKS | ANCHORED_BLOCKS
queue_position
:
position in the replacement queue
attribute_specifications
:
whether, after the square-bracketed link text,
there may be CMD attribute specifications supplied,
and if so, what the default specification is
prohibited_content
:
whether block tags or anchored block tags are prohibited
in the square-bracketed link text and the square-bracketed label
Defines a replacement rule for referenced links.
(A generalisation of the replacement that processes
[«link text»][«label»]
in Markdown.)
There are many instances in which the result of a replacement should not be altered further by replacements to follow. To protect a string from further alteration, it is temporarily replaced by a placeholder consisting of code points in the main Unicode Private Use Area.
Specifically, the placeholder for a string is of the following form:
«marker»«run_characters»«marker»
«marker»
is «U+F8FF»
.
«run_characters»
are between «U+E000»
and «U+E0FF»
each representing a Unicode byte of the string.
It is assumed that the user will not define replacement rules that
tamper with strings of the form
«marker»«run_characters»«marker»
.
£3
.
U+00A3
and U+0033
.
\xC2\xA3
and \x33
.
«run_characters»
are therefore
«U+E0C2»«U+E0A3»«U+E033»
.
«U+F8FF»«U+E0C2»«U+E0A3»«U+E033»«U+F8FF»
.
When a CMD replacement rule is defined with
attribute_specifications
not equal to NONE
,
it allows HTML attributes to be specified by CMD attribute specifications
enclosed in curly brackets.
CMD attribute specifications may be of the following forms:
«name»="«quoted value (whitespace allowed)»"
«name»=«bare-value»
#«id»
.«class»
r«rowspan»
c«colspan»
w«width»
h«height»
-«delete-name»
«boolean-name»
In the two forms with an explicit equals sign,
the following abbreviations are allowed for «name»
:
#
for id
.
for class
l
for lang
r
for rowspan
c
for colspan
w
for width
h
for height
s
for style
#inline-code
:
`{#foo .bar l=en-AU title="baz"} test`
<code id="foo" class="bar" lang="en-AU" title="baz">test</code>
`{} test`
<code>test</code>
class
values will supersede earlier ones:
`{#1 #2 title=A title=B} test`
<code id="2" title="B">test</code>
class
values will accumulate:
`{.a .b class=c .d} test`
<code class="a b c d">test</code>
class
to reset it:
`{.a .b -class class=c .d} test`
<code class="c d">test</code>